- C++ Slot Machine Console Game
- C++ Slot Machine Console Machine

Slot machine has 3 wheels each with 7 positions, as described before 2. Program must ask user how many coins are being inserted, either 1, 2, or 3. Any number outside of range ends the program, and 0 is to exit program.
C++ Slot Machine Console Game
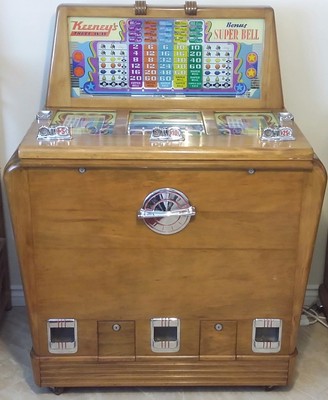
C++ Slot Machine Console Machine
P: 8 | I need some help. I am getting an error of: local function definitions are illegal. What does this mean? Can anybody help me out a little? Thank you. //Specification: This program simulates a three //wheeled slot machine. Each wheel will randomly //display three numbers. When the numbers on the //wheels match the user is award points. The Slot //machine requires the user to enter tokens to play. #include <iostream> #include <ctime> using std::cout; using std::cin; using std::endl; class slotMachine { private: int wheelA; int wheelB; int wheelC; double payOut; //amount of $ won during the current turn double moneyInMachine; //total amount of $ in the machine double playersBalance; //the amount of $ not yet played double gameCost; //the cost of one pull double moneyPaid; //the money put in by user public: //prototypes slotMachine(); bool displayMenu(void); bool pullHandle(void); void spinWheel(int &); double calculatePayout(); void insertCoin(double ); void displaySpinResults(); int Random(int, int); void displayTotals(); }; int main(void) { //create a slot machine object slotMachine mySlot; //Start the slot machine game //Keep running until the user //decides to quit bool ok = true; while (ok){ ok = mySlot.displayMenu(); } return 0; } slotMachine::slotMachine () { //constructor, set the initial state //of all the properties srand((int) time(0)); moneyInMachine = 100; moneyPaid = 0; payOut = 0; wheelA = 0; wheelB = 0; wheelC = 0; gameCost = 1; } bool slotMachine::displayMenu(void){ //main menu, executes the command selected by the //user or returns a value to terminate game execution //variable int choice = 0; //declare variables char usersChoice = 'E'; bool continueGame = true; //display menu opitions cout << 'Welcome to Las Vegas Casino n'; cout << 'Please choose an option: n'; cout << '(E)nd, (P)ull, P(A)Y, (T)otals'; //get the input cin >> usersChoice; switch (usersChoice){ case 'E': //end game continueGame = false; break; case 'A': //pay continueGame = true; //prompt user to pay cout << 'Please enter $1.00. n' << gameCost << endl; //get input cout << 'Thank you for entering your money. n' << moneyPaid << endl; break; case 'P': //user pulls the handle continueGame = true; if (pullHandle()){ displaySpinResults(); calculatePayout(); break; case 'T': //show the totals continueGame = true; displayTotals(); break; } return continueGame; } bool slotMachine::pullHandle(void){//local function defintions are illegal //checks to see if there is money //given by user then assigns a random value //to each wheel. Deducts the cost of one //pull from the users money. double moneyInMachine = 100; int wheelA = 1; int wheelB = 2; int wheelC = 3; moneyInMachine = moneyInMachine - moneyPaid; cout << 'You have n' << moneyInMachine << endl; return true; } void slotMachine::spinWheel(int &theWheel){//local function definitions are illegal //assign a random value to a wheel int wheelA = 0; int wheelB = 0; int wheelC = 0; wheelA; { wheelA = 1 + rand() % (3 - 1 + 1); } wheelB; { wheelB = 1 + rand() % (3 - 1 + 1); } wheelC; { wheelC = 1 + rand() % (3 - 1 + 1); } } double slotMachine::calculatePayout(){// local function defintions are illegal //decides if the current wheel values merit a //payout and how much that payout should be. //deducts the total payout from the total //amount of money in the machine int jackPot = 1000; int goodJob = 10; int youLose = -5; int wheelA = 0; int wheelB = 0; int wheelC = 0; wheelA wheelB && wheelA wheelC; { cout << 'Jackpot!!!' << jackPot << endl; } wheelA wheelB || wheelA wheelC || wheelB wheelC; { cout << 'Good Job' << goodJob << endl; } jackPot; { moneyInMachine = moneyInMachine + jackPot; } goodJob; { moneyInMachine = moneyInMachine + goodJob; } return moneyInMachine; } void slotMachine::insertCoin(double amount = 0.0){ //local function definitions are illegal //adds to the amount of money paid by the user //adds to the total money in the machine int moneyInMachine = 100; int moneyPaid = 0; moneyInMachine = moneyPaid + moneyInMachine; } void slotMachine::displaySpinResults(){ //displays the value of the three wheels int wheelA = 0; int wheelB = 0; int wheelC = 0; cout << 'First wheel shows: n' << wheelA << endl; cout << 'Second wheel shows: n' << wheelB << endl; cout << 'Third wheel shows: n' << wheelC << endl; } void slotMachine::displayTotals(){ //displays the total money in the machine and the number //of pulls the user has left cout << 'You have this much money left: $' << moneyInMachine << endl; cout << 'You have this many turns left: ' << endl; } int slotMachine::Random(int lowerLimit, int upperLimit) { //returns a random number within the given boundary return 1 + rand() % (upperLimit - lowerLimit + 1); } } |
|